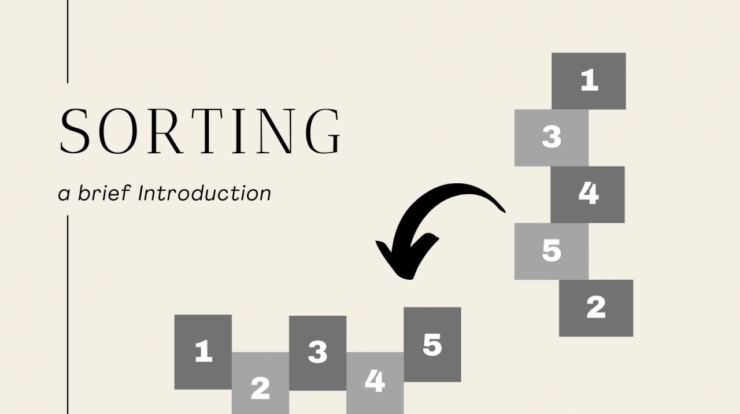
Sort definition – The concept of sorting, a fundamental operation in computer science, involves organizing data in a specific order. From simple everyday tasks to complex scientific computations, sorting plays a crucial role in managing and interpreting information effectively.
Sorting algorithms, such as bubble sort, insertion sort, and merge sort, employ different techniques to arrange data in ascending or descending order. These algorithms vary in their time and space complexities, making them suitable for specific applications.
Sort Definition
Sorting is the process of arranging data in a specific order. This can be done based on one or more criteria, such as numerical value, alphabetical order, or date.
For example, a list of numbers can be sorted in ascending or descending order, a list of names can be sorted alphabetically, and a list of dates can be sorted chronologically.
Types of Sorting
There are various sorting algorithms, each with its own time and space complexity. Some common sorting algorithms include:
- Bubble Sort
- Insertion Sort
- Merge Sort
Algorithm Name | Time Complexity | Space Complexity |
---|---|---|
Bubble Sort | O(n^2) | O(1) |
Insertion Sort | O(n^2) | O(1) |
Merge Sort | O(n log n) | O(n) |
Applications of Sorting
Sorting is widely used in various applications, including:
- Organizing data for efficient retrieval and analysis
- Searching for specific information in a large dataset
- Optimizing processes by arranging data in a logical order
- Data analysis and visualization
- Database management
- Computer graphics
Implementation
Sorting algorithms can be implemented in various programming languages. Here’s an example of bubble sort in Python:
def bubble_sort(array):
for i in range(len(array)):
for j in range(0, len(array)
- i
- 1):
if array[j] > array[j + 1]:
array[j], array[j + 1] = array[j + 1], array[j]
return array
Performance Analysis
The performance of sorting algorithms depends on factors such as the data size and the algorithm choice. The following table provides the time complexities of some common sorting algorithms:
Algorithm Name | Best-Case Time Complexity | Average-Case Time Complexity | Worst-Case Time Complexity |
---|---|---|---|
Bubble Sort | O(n) | O(n^2) | O(n^2) |
Insertion Sort | O(n) | O(n^2) | O(n^2) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) |
Advanced Sorting Techniques, Sort definition
There are advanced sorting techniques that offer improved performance for specific scenarios. These techniques include:
- Quicksort
- Radix Sort
Quicksort is a divide-and-conquer algorithm with an average time complexity of O(n log n) and a worst-case time complexity of O(n^2).
Radix sort is a non-comparative sorting algorithm that sorts data based on individual digits or bits. It has a time complexity of O(n – k), where k is the number of digits or bits in the data.
Optimization
Sorting algorithms can be optimized using various techniques, such as:
- Using specialized data structures like balanced trees or hash tables
- Parallelizing the sorting process
These optimizations can significantly improve the performance of sorting algorithms, especially for large datasets.
Applications in Computer Science
Sorting is used in various areas of computer science, including:
- Databases: Sorting data in databases optimizes query performance.
- Machine Learning: Sorting is used in algorithms like decision trees and k-nearest neighbors.
- Artificial Intelligence: Sorting is used in AI techniques like genetic algorithms and neural networks.
Last Point: Sort Definition
In conclusion, sorting is an essential tool in various fields, enabling efficient data management, search operations, and optimization processes. Understanding the different sorting techniques and their applications empowers developers and researchers to harness the power of sorting for solving complex problems.
FAQs
What is the purpose of sorting?
Sorting arranges data in a specific order, making it easier to search, compare, and process information.
Which sorting algorithm is most efficient?
The efficiency of a sorting algorithm depends on the size and type of data. Merge sort and quicksort are generally considered efficient for large datasets.
How is sorting used in real-world applications?
Sorting is used in databases, search engines, data analysis, and scientific computing, among other applications.